Home>Production & Technology>Sound Effects>How To Play Sound Effects Java
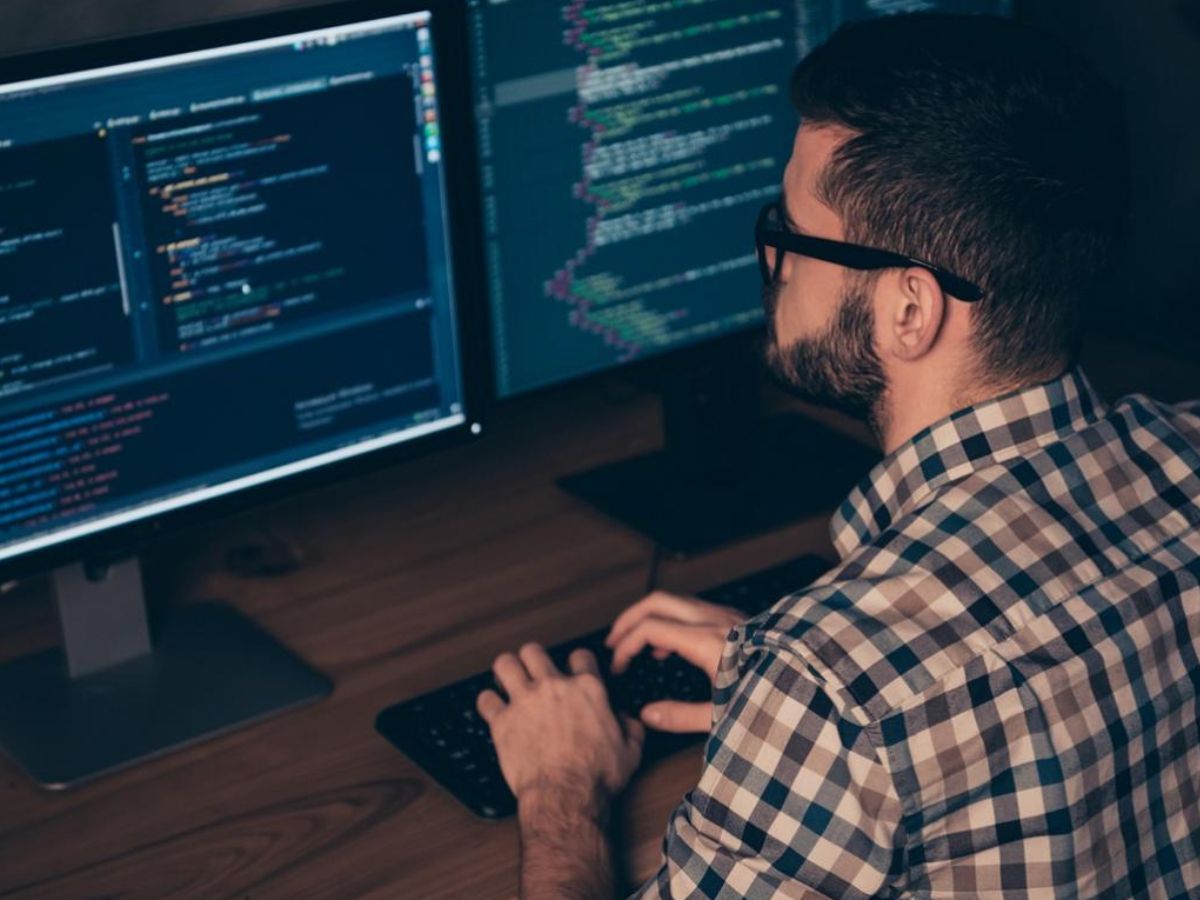
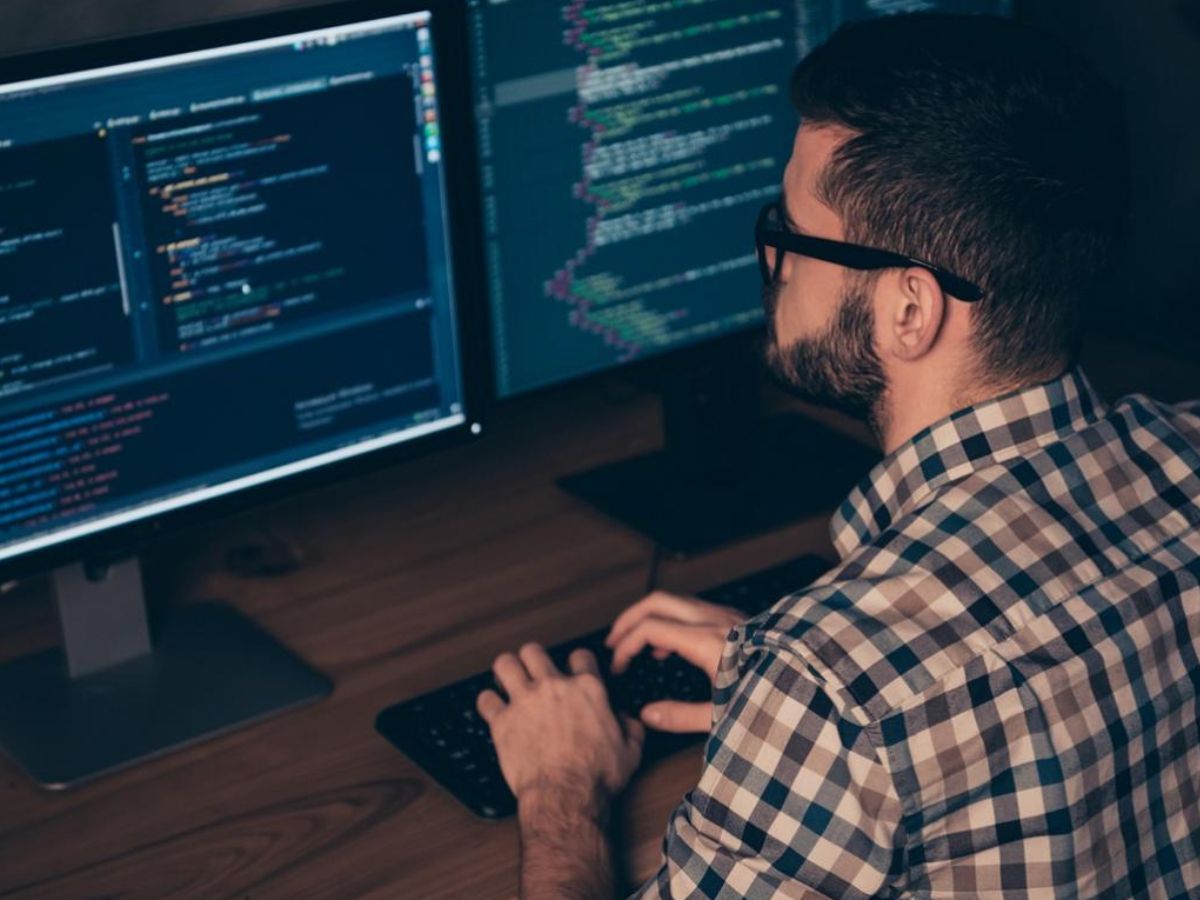
Sound Effects
How To Play Sound Effects Java
Published: November 9, 2023
Learn how to play sound effects in Java and enhance your applications. Explore different techniques and libraries for adding immersive sound effects.
(Many of the links in this article redirect to a specific reviewed product. Your purchase of these products through affiliate links helps to generate commission for AudioLover.com, at no extra cost. Learn more)
Table of Contents
- Introduction
- Overview of Sound Effects in Java
- Setting Up the Development Environment
- Playing Sound Effects with Java
- Creating and Loading Sound Files
- Playing Sound Effects Using java.awt.Toolkit
- Playing Sound Effects Using javax.sound.sampled.Clip
- Playing Sound Effects Using javax.sound.sampled.SourceDataLine
- Conclusion
Introduction
Welcome to the world of sound effects in Java! Sound effects can add a whole new dimension to your Java applications, whether you’re developing a game, a multimedia project, or any other software that requires audio enhancements. By adding sound effects, you can create a more immersive and engaging experience for your users.
In this article, we will dive into the world of sound effects in Java and explore how you can incorporate them into your projects. We’ll cover different methods and libraries you can use to play sound effects, as well as the steps you need to follow to set up your development environment properly.
Sound effects can range from simple notification sounds to complex audio compositions. They can be used to provide feedback, indicate events, create atmosphere, or simply enhance the overall user experience. Whether you need explosions, footsteps, music, or any other type of sound, Java provides various tools and libraries to help you achieve your desired effects.
Whether you’re a beginner or an experienced Java developer, this article will guide you through the process of playing sound effects in Java. We’ll cover all the necessary steps, from creating and loading sound files to playing them using different methods. By the end of this article, you’ll have a solid understanding of how to incorporate sound effects into your Java applications.
So, put on your headphones and get ready to explore the exciting world of sound effects in Java!
Overview of Sound Effects in Java
Before we dive into the technical details of playing sound effects in Java, let’s first take a moment to understand the key concepts and components involved.
In Java, sound effects are typically represented as audio files in various formats such as WAV, MP3, or OGG. These files contain the actual audio data that will be played back to create the desired sound effect. Java provides several libraries and classes that allow you to load, manipulate, and play these sound files.
One of the main libraries for working with sound effects in Java is the Java Sound API. This API provides a set of classes and methods that enable you to play, control, and synthesize audio. It allows you to work with different audio formats, control volume, play sounds asynchronously, and more.
In addition to the Java Sound API, there are other third-party libraries available that provide advanced features for working with sound effects in Java. Some popular libraries include JOAL (Java Bindings for OpenAL), TinySound, and JLayer.
When working with sound effects in Java, it’s important to consider the different approaches for playing the audio. You can use classes such as java.awt.Toolkit, javax.sound.sampled.Clip, and javax.sound.sampled.SourceDataLine to play sound effects. Each approach has its own advantages and limitations, so it’s essential to choose the right method based on your specific requirements.
Sound effects can be a critical element in different types of applications. In games, they can provide feedback and enhance the overall gaming experience by simulating realistic sounds. In multimedia applications, sound effects can be used to synchronize audio with visual content to create immersive experiences. They are also commonly used in user interfaces to provide audio feedback for user actions and events.
Now that we have a better understanding of the role of sound effects in Java and the key components involved, let’s move on to setting up our development environment to start working with sound effects.
Setting Up the Development Environment
Before we can start playing sound effects in Java, we need to set up our development environment with the necessary tools and libraries. Here are the steps you need to follow:
- Install Java Development Kit (JDK): Make sure you have the latest version of JDK installed on your machine. You can download it from the official Oracle website and follow the installation instructions specific to your operating system.
- Choose an Integrated Development Environment (IDE): While Java can be developed using a text editor and command-line tools, using an IDE can significantly improve your productivity. Some popular choices include Eclipse, IntelliJ IDEA, and NetBeans. Install your preferred IDE and set it up according to the provided instructions.
- Import Required Libraries: Depending on the method you choose for playing sound effects, you might need to import additional libraries into your project. For example, if you’re using the Java Sound API, no additional imports are required since it is part of the standard Java libraries. However, if you’re using a third-party library like JOAL or TinySound, you’ll need to include those libraries in your project and ensure they are properly configured.
- Prepare Sound Files: Gather the sound files you want to use as sound effects in your Java application. Make sure the files are in a compatible audio format, such as WAV, MP3, or OGG. Place the sound files in a designated folder within your project structure for easy access.
- Test Environment Setup: Once you have completed the above steps, it’s a good practice to test your development environment setup. Create a simple Java program that loads and plays a sound file to ensure everything is functioning correctly. This will also help you identify any potential issues or missing dependencies.
With your development environment set up and ready to go, you can now proceed to the next section, where we will explore different methods for playing sound effects in Java.
Playing Sound Effects with Java
In Java, there are multiple methods and libraries available for playing sound effects. The choice of method depends on the specific requirements of your application and the level of control you need over the playback. Let’s explore three common methods for playing sound effects in Java:
1. Using java.awt.Toolkit
The java.awt.Toolkit
class provides a simple way to play short sound clips. This method is suitable for playing small sound effects, such as button clicks or notification sounds. The Toolkit
class provides a static method called getDefaultToolkit()
that returns an instance of the Toolkit
class. You can use the getSound
method of the Toolkit
class to load and play a sound file.
2. Using javax.sound.sampled.Clip
The javax.sound.sampled.Clip
class provides a more advanced approach to playing sound effects in Java. This class allows you to load audio files, control playback, and apply various effects. You can create an instance of the Clip
class using the AudioSystem
class and load the sound file using the open
method. You can then use methods like start
, stop
, and loop
to control the playback.
3. Using javax.sound.sampled.SourceDataLine
The javax.sound.sampled.SourceDataLine
class provides the most low-level and customizable option for playing sound effects in Java. It allows you to directly access and manipulate the audio data, offering granular control over playback. You can obtain an instance of the SourceDataLine
class using the AudioSystem
class and use methods like write
and drain
to control the playback and manage the audio data.
Each method has its advantages and limitations, so it’s important to choose the one that best suits your needs. The java.awt.Toolkit
method is the simplest to implement but offers limited control. The javax.sound.sampled.Clip
method provides more control over playback, while the javax.sound.sampled.SourceDataLine
method offers the most flexibility but requires a deeper understanding of audio manipulation.
Now that we have an overview of the different methods available for playing sound effects in Java, let’s move on to the next sections, where we will explore how to create and load sound files for use in our applications.
Creating and Loading Sound Files
In order to play sound effects in Java, we first need to create or obtain sound files that will be used for the audio playback. Sound files can be in various audio formats such as WAV, MP3, or OGG. Here are the steps to create and load sound files:
- Create Sound Files: If you need custom sound effects, you can create them using audio editing software or record them using a microphone. Make sure to save the sound files in a supported format and consider the quality and compatibility with your target platform.
- Acquire Sound Files: If you don’t have the tools or resources to create custom sound effects, you can acquire them from various sources. There are many royalty-free sound libraries available online that offer a wide range of sound effects that you can use in your Java applications. Just make sure to read and comply with the licensing terms of the sound files you choose.
- Load Sound Files in Java: Once you have your sound files ready, you need to load them into your Java application. The method of loading depends on the library or approach you are using to play sound effects. For example, with the
java.awt.Toolkit
method, you can use theToolkit
class’sgetSound
method to load a sound file by providing its file path. - Store Sound Files: It’s a good practice to organize and store your sound files in a designated folder within your project structure. This makes it easier to manage and access the sound files when needed. Consider creating separate folders for different categories of sound effects to keep everything organized.
It’s important to choose suitable sound files that match the purpose of your application and enhance the overall user experience. Consider factors such as the theme, mood, and desired impact of the sound effects. Experiment with different sound files and variations to find the perfect fit.
By following these steps, you can create or obtain sound files and load them into your Java application to be used as sound effects. In the next sections, we’ll explore how to play sound effects using various Java libraries and classes.
Playing Sound Effects Using java.awt.Toolkit
The java.awt.Toolkit
class provides a convenient and straightforward way to play short sound effects in Java. This method is suitable for playing small audio clips, such as button clicks or notification sounds.
Here are the steps to play sound effects using java.awt.Toolkit
:
- Obtain the Default Toolkit: Start by getting an instance of the default
java.awt.Toolkit
using thegetDefaultToolkit()
method: - Load the Sound File: Use the
getSound()
method of theToolkit
class to load the sound file by providing its file path as a parameter: - Play the Sound: Call the
play()
method on theAudioClip
object to play the loaded sound file: - Stop the Sound: If you need to stop the sound before it finishes playing, you can call the
stop()
method on theAudioClip
object:
java
Toolkit toolkit = Toolkit.getDefaultToolkit();
java
URL soundURL = getClass().getResource(“path/to/sound/file.wav”);
AudioClip audioClip = toolkit.getAudioClip(soundURL);
java
audioClip.play();
java
audioClip.stop();
The java.awt.Toolkit
method is simple and suitable for playing short sound effects. However, it has some limitations. It does not provide fine-grained control over the audio playback, such as adjusting volume or looping. Additionally, it may not support all audio formats, so make sure your sound files are compatible.
Remember to handle potential exceptions when using the java.awt.Toolkit
method for playing sound effects, such as handling NullPointerException
in case the sound file is not found.
Now that we have covered the java.awt.Toolkit
method, let’s explore other methods for playing sound effects in Java using the javax.sound.sampled.Clip
and javax.sound.sampled.SourceDataLine
classes.
Playing Sound Effects Using javax.sound.sampled.Clip
The javax.sound.sampled.Clip
class in Java provides a more advanced approach to playing sound effects. It allows for more control over audio playback and provides features such as pausing, looping, and volume adjustment. Here’s how you can use Clip
to play sound effects:
- Create a
Clip
Instance: Start by creating an instance of theClip
class using theAudioSystem
class: - Load the Sound File: Use the
open()
method of theClip
object to load the sound file, which can be in WAV, MP3, or other compatible formats: - Play the Sound: Start playing the loaded sound file by calling the
start()
method on theClip
object: - Control Playback: You can control the playback of the sound effect by using various methods of the
Clip
class. For example, you can pause the playback usingclip.stop()
, resume usingclip.start()
, or loop the sound endlessly usingclip.loop(Clip.LOOP_CONTINUOUSLY)
. You can also adjust the volume usingFloatControl
methods likesetValue()
orgetValue()
. - Release Resources: Once you are done playing the sound effect, it’s important to release the system resources associated with the
Clip
by calling theclose()
method:
java
Clip clip = AudioSystem.getClip();
java
File soundFile = new File(“path/to/sound/file.wav”);
AudioInputStream audioInputStream = AudioSystem.getAudioInputStream(soundFile);
clip.open(audioInputStream);
java
clip.start();
java
clip.close();
The javax.sound.sampled.Clip
method provides more flexibility and control over sound effects. You can manipulate the playback, adjust volume, and apply looping or other effects. However, keep in mind that the exact features and capabilities may vary depending on the specific audio format and platform compatibility.
In the next section, we’ll explore another method for playing sound effects in Java using the javax.sound.sampled.SourceDataLine
class, which offers even more low-level control.
Playing Sound Effects Using javax.sound.sampled.SourceDataLine
The javax.sound.sampled.SourceDataLine
class in Java provides the most low-level and customizable option for playing sound effects. It allows direct access to the audio data, giving you granular control over playback. Here’s how you can use SourceDataLine
to play sound effects:
- Create a
SourceDataLine
Instance: Start by creating an instance of theSourceDataLine
class using theAudioSystem
class: - Open the Line: Use the
open()
method of theSourceDataLine
object to open the audio line: - Start the Line: Start the line for playback by calling the
start()
method: - Write Audio Data: Write the audio data to the line using the
write()
method. You’ll need to convert your sound file into a suitable byte array (audio data) before writing it: - Control Playback: Similar to the other methods, you can control the playback by pausing with
line.stop()
, resuming withline.start()
, or tweaking the volume usingFloatControl
methods. - Drain and Close the Line: Once you’re done playing the sound effect, drain the line, ensuring that all pending data is played, and close the line to release system resources:
java
SourceDataLine line = AudioSystem.getSourceDataLine(null);
java
AudioFormat audioFormat = new AudioFormat(sampleRate, sampleSizeInBits, channels, signed, bigEndian);
line.open(audioFormat);
java
line.start();
java
byte[] audioData = // convert your sound file into byte array
line.write(audioData, 0, audioData.length);
java
line.drain();
line.close();
The javax.sound.sampled.SourceDataLine
method provides the most flexibility and control over sound effects. It allows you to manipulate audio data directly, giving you precise control over playback. However, it requires a deeper understanding of audio manipulation and may involve more complex code compared to the previous methods.
With these methods explained, you can now choose the approach that best suits your needs for playing sound effects in Java. Experiment with different methods, adjust playback settings, and explore different libraries to create engaging and immersive audio experiences in your applications.
In the next section, we’ll conclude this article by summarizing the key points and highlighting the benefits of incorporating sound effects into your Java projects.
Conclusion
Congratulations! You’ve now learned how to incorporate sound effects into your Java projects. By adding sound effects, you can enhance user experiences, create immersive environments, and provide valuable feedback in your applications. Let’s summarize the key points we’ve covered in this article:
– Sound effects in Java can be used to enhance games, multimedia projects, user interfaces, and various other software applications.
– Java provides different libraries and classes for playing sound effects, such as java.awt.Toolkit
, javax.sound.sampled.Clip
, and javax.sound.sampled.SourceDataLine
.
– The choice of method depends on the specific requirements you have for your application, such as simplicity, control, or customizability.
– When setting up your development environment, ensure you have the latest version of JDK installed, choose an IDE, and import any necessary libraries for sound playback.
– Create or acquire sound files in compatible formats such as WAV, MP3, or OGG, and load them into your Java application for playback.
– Play sound effects using the appropriate methods and classes, adjusting volume, looping, and controlling playback as desired.
– Remember to release system resources and handle exceptions accordingly to ensure smooth execution and error-free playback.
By successfully implementing sound effects in your Java applications, you can make them more engaging, immersive, and enjoyable for your users. The choice of sound effects and their integration into your software can greatly impact the overall user experience. Therefore, take the time to choose and create sound effects that align with your application’s purpose and desired atmosphere.
Don’t be afraid to experiment, iterate, and fine-tune your sound effects to achieve the desired results. Additionally, continue exploring the Java Sound API and other related libraries to broaden your knowledge and enhance your sound effect capabilities.
Now, armed with your newfound expertise, go forth and elevate your Java projects with captivating and dynamic sound effects!